7 CSS Tricks Every Developer Should Know
CSS is full of clever techniques that can solve common design challenges. Here are seven CSS tricks that every web developer should have in their toolkit. These solutions are both elegant and practical, working across modern browsers.
1. Center Anything Both Vertically and Horizontally
.center-perfect {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh; /* Optional: full viewport height */
}
/* Alternative Grid approach */
.center-grid {
display: grid;
place-items: center;
}
2. Smooth Scrolling Without JavaScript
html {
scroll-behavior: smooth;
}
/* Use with anchor links */
<a href="#section-2">Go to Section 2</a>
<div id="section-2">Section 2 Content</div>
3. Custom Text Selection Color
::selection {
background: #ffb7b7;
color: #333333;
}
/* For Firefox */
::-moz-selection {
background: #ffb7b7;
color: #333333;
}
4. Truncate Text with Ellipsis
.truncate {
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
max-width: 200px; /* Adjust as needed */
}
/* Multi-line truncation */
.truncate-multiline {
display: -webkit-box;
-webkit-line-clamp: 3; /* Number of lines */
-webkit-box-orient: vertical;
overflow: hidden;
}
5. Custom Scrollbar Styling
.custom-scrollbar {
/* Width */
::-webkit-scrollbar {
width: 10px;
}
/* Track */
::-webkit-scrollbar-track {
background: #f1f1f1;
border-radius: 5px;
}
/* Handle */
::-webkit-scrollbar-thumb {
background: #888;
border-radius: 5px;
}
/* Handle on hover */
::-webkit-scrollbar-thumb:hover {
background: #555;
}
}
6. Responsive Aspect Ratio Boxes
.aspect-ratio-box {
aspect-ratio: 16 / 9;
width: 100%;
background: #f0f0f0;
}
/* Legacy approach for older browsers */
.aspect-ratio-box-legacy {
position: relative;
padding-top: 56.25%; /* 16:9 Aspect Ratio */
}
.aspect-ratio-box-legacy > * {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
7. CSS-only Tooltip
.tooltip {
position: relative;
display: inline-block;
}
.tooltip:before {
content: attr(data-tooltip);
position: absolute;
bottom: 100%;
left: 50%;
transform: translateX(-50%);
padding: 5px 10px;
background: #333;
color: white;
border-radius: 4px;
font-size: 14px;
white-space: nowrap;
opacity: 0;
visibility: hidden;
transition: opacity 0.3s ease;
}
.tooltip:hover:before {
opacity: 1;
visibility: visible;
}
/* Usage: <div class="tooltip" data-tooltip="Hello!">Hover me</div> */
These CSS tricks demonstrate the power of modern CSS and can significantly reduce the need for JavaScript in many common scenarios. They're well-supported across modern browsers and can be easily customized to match your project's design system. Remember to always check browser compatibility when implementing these solutions in production environments.
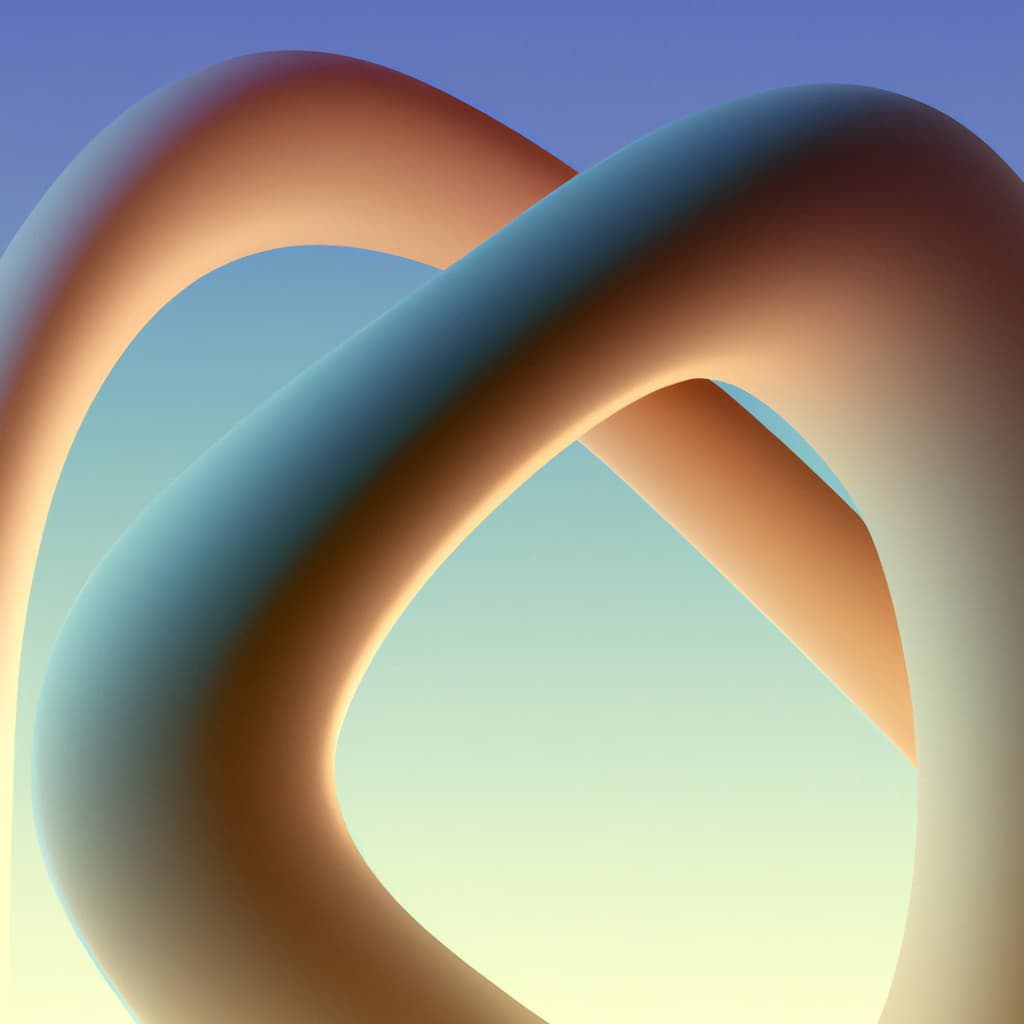