Essential Semantic HTML Tags You Should Be Using
Semantic HTML is crucial for accessibility, SEO, and maintaining clean, meaningful code. While many developers default to generic <div> and <span> elements, HTML5 provides a rich set of semantic tags that can make your markup more meaningful and accessible. Let's explore some of the most useful semantic tags you might not be using.
1. The <article> Tag - Perfect for Self-Contained Content
<article class="blog-post">
<h2>Post Title</h2>
<p class="metadata">Posted by <address class="author">Sarah Smith</address></p>
<p>Article content goes here...</p>
<footer>
<p>Comments: 23 | Likes: 45</p>
</footer>
</article>
2. The <figure> and <figcaption> Tags - Perfect for Images, Diagrams, and Code Snippets
<figure>
<img src="graph.png" alt="Sales data for Q3 2024">
<figcaption>
Fig.1 - Quarterly sales showing 25% growth in Q3 2024
</figcaption>
</figure>
<!-- Also great for code examples -->
<figure>
<pre><code>
const greeting = "Hello, World!";
</code></pre>
<figcaption>Example 1: Basic JavaScript greeting</figcaption>
</figure>
3. The <time> Tag - Semantic Way to Display Dates and Times
<p>The meeting is scheduled for
<time datetime="2024-01-15T14:30:00">
January 15th at 2:30pm
</time>
</p>
<p>Store hours:
<time datetime="09:00">9am</time> to
<time datetime="17:00">5pm</time>
</p>
<!-- For durations -->
<time datetime="PT2H30M">2 hours and 30 minutes</time>
4. The <details> and <summary> Tags - Native Accordion Implementation
<details>
<summary>Click to view more information</summary>
<p>This content is hidden by default and shows when clicked.
Perfect for FAQs, documentation, and expandable content.</p>
<ul>
<li>No JavaScript required</li>
<li>Built-in accessibility</li>
<li>Native animations</li>
</ul>
</details>
5. The <mark> Tag - Highlight Text Semantically
<p>Search results for "semantic HTML":
Here's an article about <mark>semantic HTML</mark> and how
<mark>semantic</mark> tags improve accessibility.
</p>
<style>
mark {
background-color: #fff3bf;
padding: 0 2px;
border-radius: 2px;
}
</style>
6. The <aside> Tag - Related but Separate Content
<article>
<h1>Main Article Title</h1>
<p>Main content goes here...</p>
<aside>
<h3>Related Articles</h3>
<ul>
<li><a href="#">Related topic 1</a></li>
<li><a href="#">Related topic 2</a></li>
</ul>
</aside>
</article>
7. The <dialog> Tag - Native Modal Windows
<dialog id="favoriteDialog">
<h2>Choose your favorite color</h2>
<form method="dialog">
<button value="blue">Blue</button>
<button value="red">Red</button>
<button value="green">Green</button>
</form>
</dialog>
<button onclick="favoriteDialog.showModal()">
Open Color Picker
</button>
Using semantic HTML not only makes your code more readable and maintainable but also provides valuable context to browsers, search engines, and assistive technologies. These tags come with built-in accessibility features and appropriate default styles, reducing the need for extra ARIA roles and custom styling in many cases.
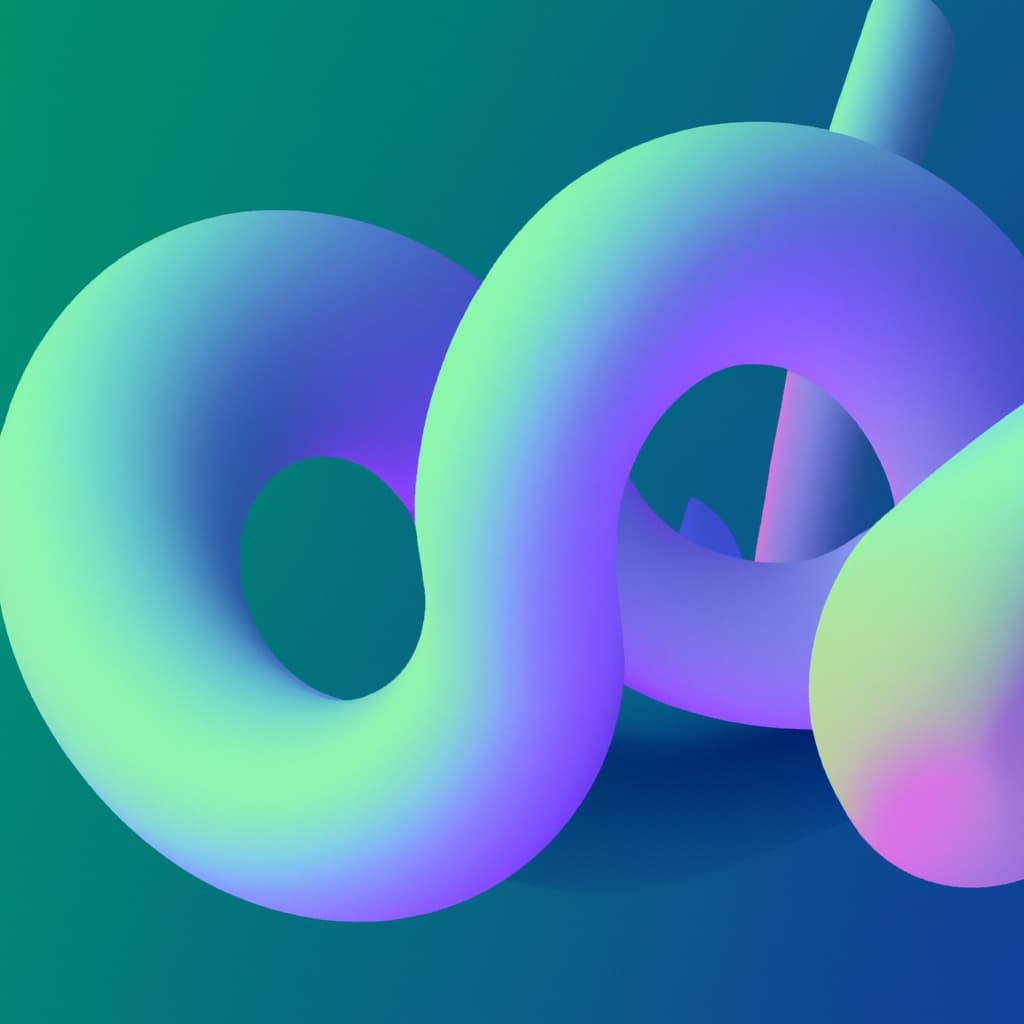