Setting up a Three.js Scene: A Beginner's Guide
Three.js is a powerful JavaScript library that makes it easy to create and display 3D graphics in a web browser. In this guide, we'll walk through setting up a basic Three.js scene from scratch, including essential components like the canvas, renderer, and camera. Whether you're new to 3D graphics or looking to refresh your knowledge, this tutorial will give you a solid foundation.
npm install three
npm install --save-dev @types/three
Before diving into the Three.js implementation, we need to set up our HTML structure. This will serve as the container for our 3D scene. The key here is to create a full-width canvas that will house our 3D graphics, with proper styling to ensure it fills the viewport.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Three.js Scene</title>
<style>
body { margin: 0; }
canvas { display: block; }
</style>
</head>
<body>
<script type="module" src="./dist/main.js"></script>
</body>
</html>
Now for the core of our application - the TypeScript implementation. We'll create a class that handles the scene setup, camera configuration, and renderer initialization. This structured approach makes our code more maintainable and easier to extend later.
import * as THREE from 'three';
class ThreeJsScene {
private scene: THREE.Scene;
private camera: THREE.PerspectiveCamera;
private renderer: THREE.WebGLRenderer;
constructor() {
// Initialize the scene
this.scene = new THREE.Scene();
// Set up the camera
this.camera = new THREE.PerspectiveCamera(
75, // Field of view
window.innerWidth / window.innerHeight, // Aspect ratio
0.1, // Near plane
1000 // Far plane
);
this.camera.position.z = 5;
// Create the renderer
this.renderer = new THREE.WebGLRenderer({
antialias: true
});
this.renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(this.renderer.domElement);
// Handle window resizing
window.addEventListener('resize', this.onWindowResize.bind(this));
// Start the animation loop
this.animate();
}
private onWindowResize(): void {
this.camera.aspect = window.innerWidth / window.innerHeight;
this.camera.updateProjectionMatrix();
this.renderer.setSize(window.innerWidth, window.innerHeight);
}
private animate(): void {
requestAnimationFrame(this.animate.bind(this));
this.renderer.render(this.scene, this.camera);
}
}
// Initialize the scene
new ThreeJsScene();
The code above sets up all the essential components for a Three.js scene. The PerspectiveCamera is configured with a 75-degree field of view and an aspect ratio matching the window dimensions. The renderer is created with antialiasing enabled for smoother edges, and we've implemented proper window resize handling to ensure our scene always looks correct regardless of viewport size. The animate method sets up the render loop using requestAnimationFrame, which will be crucial when we start adding animations and interactions to our scene.
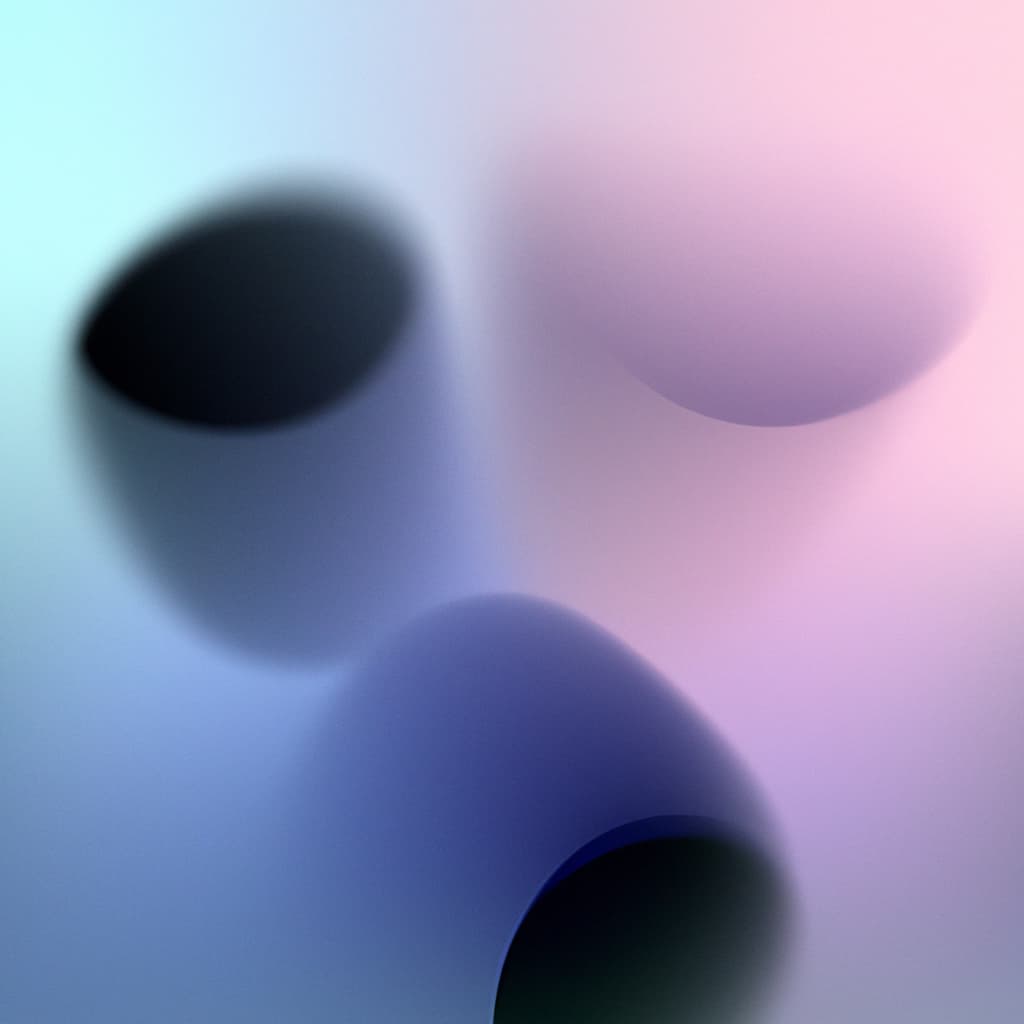